Convert Seconds To Hh Mm Ss
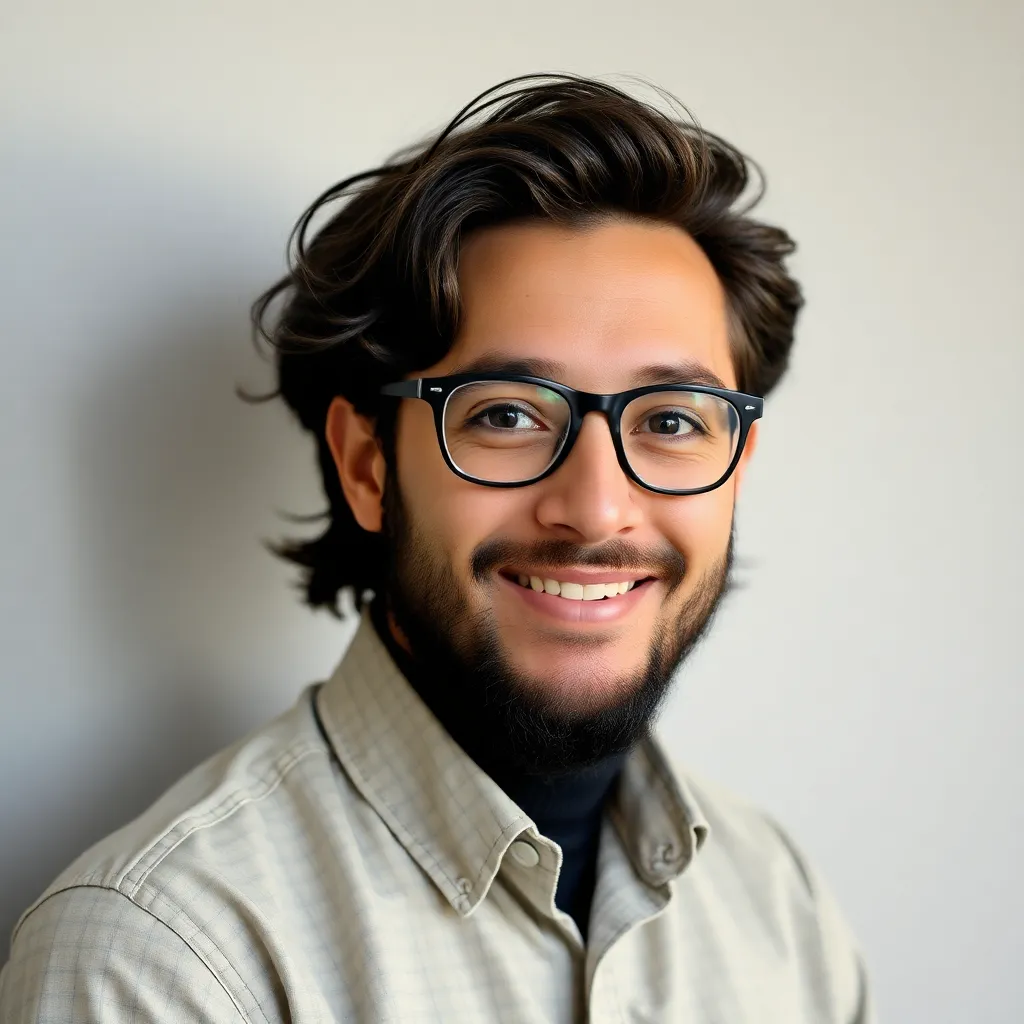
Webtuts
Apr 21, 2025 · 5 min read

Table of Contents
Convert Seconds to HH:MM:SS: A Comprehensive Guide
Converting seconds into hours, minutes, and seconds (HH:MM:SS) is a common task in various programming contexts and everyday life. Whether you're working with timestamps, calculating durations, or simply need to present a time value in a more user-friendly format, understanding this conversion is crucial. This comprehensive guide will explore different methods, provide code examples in popular programming languages, and address common challenges in handling this conversion accurately.
Understanding the Conversion Logic
The fundamental principle lies in the relationship between these time units:
- 60 seconds (s) = 1 minute (min)
- 60 minutes (min) = 1 hour (hr)
Therefore, to convert a given number of seconds into HH:MM:SS format, we need to successively divide and find the remainders.
- Hours: Divide the total seconds by 3600 (60 seconds/minute * 60 minutes/hour). The quotient represents the number of hours.
- Minutes: Obtain the remainder from the previous division (total seconds modulo 3600). Divide this remainder by 60. The quotient represents the number of minutes.
- Seconds: The remainder from the minute calculation is the number of remaining seconds.
Method 1: Using Integer Division and Modulo Operator
This is the most straightforward approach, leveraging integer division (//
in Python, /
in some languages) and the modulo operator (%
). Let's illustrate with Python code:
def seconds_to_hhmmss(seconds):
"""Converts seconds to HH:MM:SS format.
Args:
seconds: The total number of seconds.
Returns:
A string representing the time in HH:MM:SS format. Returns an error message if input is invalid.
"""
if not isinstance(seconds, int) or seconds < 0:
return "Invalid input: Seconds must be a non-negative integer."
hours = seconds // 3600
minutes = (seconds % 3600) // 60
seconds_remaining = (seconds % 3600) % 60
return "{:02d}:{:02d}:{:02d}".format(hours, minutes, seconds_remaining)
#Example Usage
total_seconds = 7500
formatted_time = seconds_to_hhmmss(total_seconds)
print(f"{total_seconds} seconds is equal to {formatted_time}")
total_seconds = -10
formatted_time = seconds_to_hhmmss(total_seconds)
print(f"{total_seconds} seconds is equal to {formatted_time}")
total_seconds = 3661
formatted_time = seconds_to_hhmmss(total_seconds)
print(f"{total_seconds} seconds is equal to {formatted_time}")
total_seconds = 86400 #One day
formatted_time = seconds_to_hhmmss(total_seconds)
print(f"{total_seconds} seconds is equal to {formatted_time}")
This Python function efficiently handles the conversion. The {:02d}
format specifier ensures that hours, minutes, and seconds are always displayed with leading zeros (e.g., 01, 02 instead of 1, 2) for consistent formatting. Crucially, error handling is included to manage invalid inputs, ensuring robustness.
Method 2: Utilizing the datetime
Module (Python)
Python's datetime
module provides a more elegant solution for time-related operations. This method is particularly useful when dealing with more complex time manipulations.
import datetime
def seconds_to_hhmmss_datetime(seconds):
"""Converts seconds to HH:MM:SS format using the datetime module.
Args:
seconds: The total number of seconds.
Returns:
A string representing the time in HH:MM:SS format. Returns an error message if input is invalid.
"""
if not isinstance(seconds, int) or seconds < 0:
return "Invalid input: Seconds must be a non-negative integer."
return str(datetime.timedelta(seconds=seconds))
# Example Usage
total_seconds = 7500
formatted_time = seconds_to_hhmmss_datetime(total_seconds)
print(f"{total_seconds} seconds is equal to {formatted_time}")
total_seconds = 3661
formatted_time = seconds_to_hhmmss_datetime(total_seconds)
print(f"{total_seconds} seconds is equal to {formatted_time}")
total_seconds = 86400 # One day
formatted_time = seconds_to_hhmmss_datetime(total_seconds)
print(f"{total_seconds} seconds is equal to {formatted_time}")
The datetime.timedelta
object simplifies the conversion. It directly handles the calculation and provides the formatted output. Note that this method implicitly handles potential overflow; it correctly calculates time spans exceeding 24 hours.
Method 3: JavaScript Implementation
JavaScript also offers a straightforward approach using similar mathematical operations:
function secondsToHHMMSS(seconds) {
if (typeof seconds !== 'number' || seconds < 0) {
return "Invalid input: Seconds must be a non-negative number.";
}
const hours = Math.floor(seconds / 3600);
const minutes = Math.floor((seconds % 3600) / 60);
const remainingSeconds = seconds % 60;
return `${hours.toString().padStart(2, '0')}:${minutes.toString().padStart(2, '0')}:${remainingSeconds.toString().padStart(2, '0')}`;
}
// Example Usage
let totalSeconds = 7500;
let formattedTime = secondsToHHMMSS(totalSeconds);
console.log(`${totalSeconds} seconds is equal to ${formattedTime}`);
totalSeconds = -10;
formattedTime = secondsToHHMMSS(totalSeconds);
console.log(`${totalSeconds} seconds is equal to ${formattedTime}`);
totalSeconds = 3661;
formattedTime = secondsToHHMMSS(totalSeconds);
console.log(`${totalSeconds} seconds is equal to ${formattedTime}`);
totalSeconds = 86400; //One day
formattedTime = secondsToHHMMSS(totalSeconds);
console.log(`${totalSeconds} seconds is equal to ${formattedTime}`);
The padStart(2, '0')
method ensures consistent two-digit formatting for all time components, enhancing readability. Similar to the Python examples, error handling is implemented to manage invalid inputs.
Method 4: C# Implementation
C# offers a concise approach using integer division and the modulo operator:
using System;
public class SecondsConverter
{
public static string ConvertSecondsToHHMMSS(int seconds)
{
if (seconds < 0)
{
return "Invalid input: Seconds must be a non-negative integer.";
}
int hours = seconds / 3600;
int minutes = (seconds % 3600) / 60;
int remainingSeconds = seconds % 60;
return $"{hours:D2}:{minutes:D2}:{remainingSeconds:D2}";
}
public static void Main(string[] args)
{
int totalSeconds = 7500;
string formattedTime = ConvertSecondsToHHMMSS(totalSeconds);
Console.WriteLine($"{totalSeconds} seconds is equal to {formattedTime}");
totalSeconds = -10;
formattedTime = ConvertSecondsToHHMMSS(totalSeconds);
Console.WriteLine($"{totalSeconds} seconds is equal to {formattedTime}");
totalSeconds = 3661;
formattedTime = ConvertSecondsToHHMMSS(totalSeconds);
Console.WriteLine($"{totalSeconds} seconds is equal to {formattedTime}");
totalSeconds = 86400; //One day
formattedTime = ConvertSecondsToHHMMSS(totalSeconds);
Console.WriteLine($"{totalSeconds} seconds is equal to {formattedTime}");
}
}
The :D2
format specifier in C# achieves the same two-digit formatting as the {:02d}
in Python and padStart
in JavaScript. The Main
method provides example usage.
Handling Large Numbers of Seconds
For extremely large numbers of seconds (representing days, weeks, or even months), the output might become excessively long. Consider adding logic to handle these scenarios. You might choose to truncate the output to a specific time unit (e.g., showing only days and hours) or adjust the formatting to improve readability.
Error Handling and Input Validation
Always include thorough error handling. Check for negative input, non-numeric values, and other potential issues that could lead to unexpected results or program crashes. Robust error handling makes your code more reliable and user-friendly.
Conclusion
Converting seconds to HH:MM:SS format is a common programming task with various approaches depending on the programming language and desired level of sophistication. This guide demonstrates multiple methods, highlighting the use of integer division, the modulo operator, and specialized time libraries. Remember to prioritize error handling and input validation for robust and reliable code. By carefully considering these aspects, you can effectively implement this conversion in your projects, ensuring accurate and user-friendly time representation. The examples provided across different programming languages will allow you to easily adapt the code to your specific needs.
Latest Posts
Latest Posts
-
How Many Days Till 15 October
Apr 22, 2025
-
How Much In A Square Of Siding
Apr 22, 2025
-
1 1 2 Cup Sugar In Grams
Apr 22, 2025
-
How Many Teaspoon Is 2 Oz
Apr 22, 2025
-
How Many Feet And Inches Are In 150 Inches
Apr 22, 2025
Related Post
Thank you for visiting our website which covers about Convert Seconds To Hh Mm Ss . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.